PlayerInput Component
빌트인 컴포넌트인 PlayerInput을 플레이어 오브젝트에 추가해서 키입력을 바로 받을 수 있다.
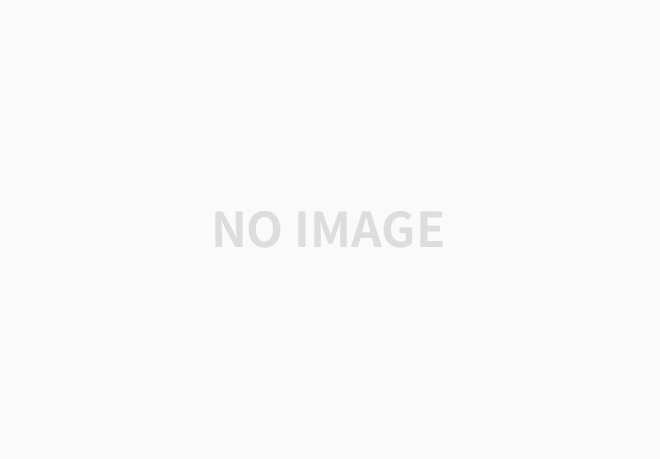
Actions에 등록된 InputSystem_Actions를 열어볼 수 있는데 일반적으로 사용되는 키로 바인딩되어 있는 걸 확인할 수 있다.
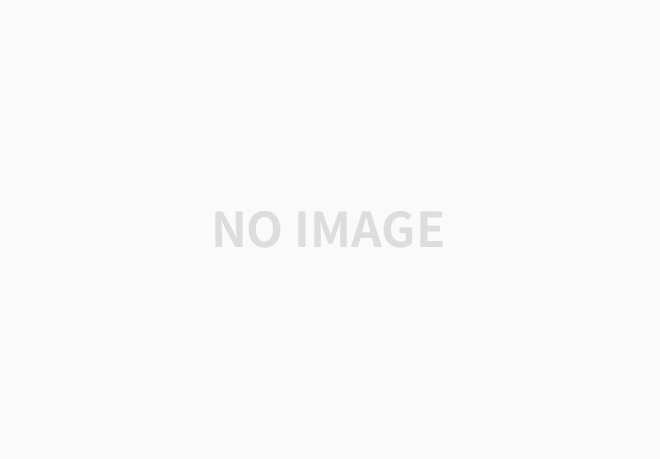
이 파일을 수정해서 바인딩 키나 값을 변경하여 처리할 수 있다.
플레이어 조작 스크립트에서 이 입력을 가져다 쓰는 방법은 다음과 같다.
private void Awake()
{
rb = GetComponent<Rigidbody>();
}
private void FixedUpdate()
{
if (currentInput != Vector2.zero)
{
// 방향 설정
body.forward = new Vector3(currentInput.x, 0, currentInput.y).normalized;
// 속도 계산
float currentSpeed = walkSpeed;
Vector3 moveVelocity = new Vector3(currentInput.x, 0, currentInput.y) * currentSpeed;
// 물리 이동
rb.linearVelocity = moveVelocity;
// 애니메이션
animator.SetFloat("Move", currentInput.magnitude);
}
else
{
// 정지 상태
animator.SetFloat("Move", 0);
rb.linearVelocity = Vector3.zero;
}
}
public void OnMove(InputValue value)
{
moveInput = value.Get<Vector2>();
}
업데이트 안에서 이동키 입력으로 변경되는 moveInput 값을 갱신해서 플레이어를 움직인다.
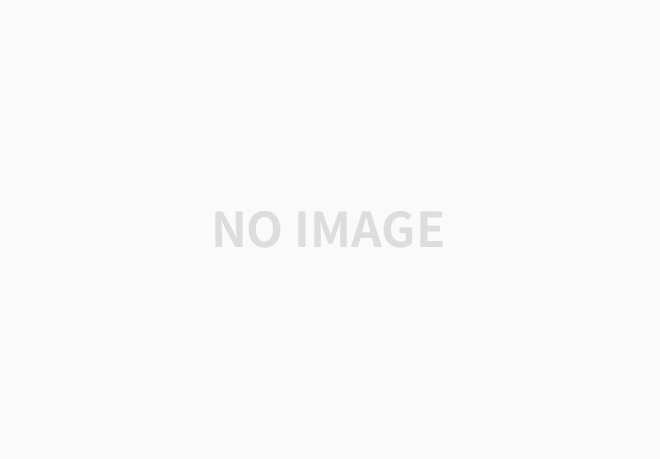
Action Properties 설정을 통해서 필요에 맞춰 수정해서 쓸 수 있다.
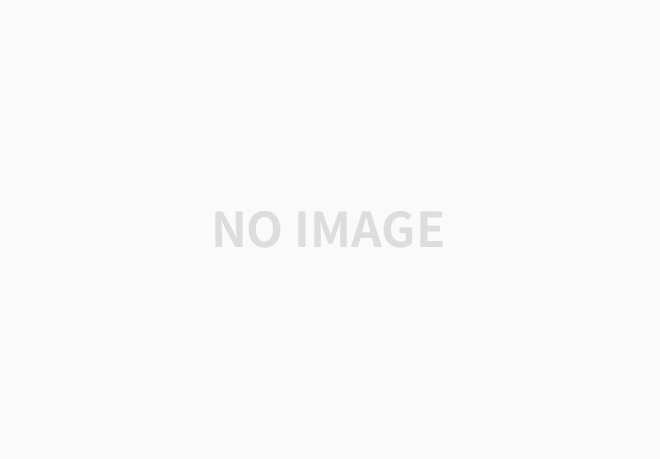
쉬프트를 누르면 달리고, 떼면 걷도록 상태를 변경하는 기능을 추가해본다.
기본 Action은 눌렀을 때만 처리하고 있는데 이 부분을 PressAndRelease로 변경한다.
그리고 Initial State Check를 활성화 해준다.
private void FixedUpdate()
{
currentInput = Vector2.SmoothDamp(
currentInput,
moveInput * (isSprint ? 1f : 0.5f),
ref smoothVelocity,
smoothTime
);
if (currentInput != Vector2.zero)
{
// 방향 설정
body.forward = new Vector3(currentInput.x, 0, currentInput.y).normalized;
// 속도 계산
float currentSpeed = isSprint ? sprintSpeed : walkSpeed;
Vector3 moveVelocity = new Vector3(currentInput.x, 0, currentInput.y) * currentSpeed;
// 물리 이동
rb.linearVelocity = moveVelocity;
// 애니메이션
animator.SetFloat("Move", currentInput.magnitude);
}
else
{
// 정지 상태
animator.SetFloat("Move", 0);
rb.linearVelocity = Vector3.zero;
}
}
private void PlayerAnimation(float moveAmount)
{
animator.SetFloat("Move", moveAmount);
}
public void OnMove(InputValue value)
{
moveInput = value.Get<Vector2>();
}
public void OnSprint(InputValue value)
{
isSprint = value.isPressed;
}
InputManager의 GetAxis처럼 입력이 서서히 -1 0 1 사이에서 움직이는 선택 없이 GetAxisRaw처럼 고정된 숫자로 값이 반환되는데 이 부분이 InputSystem에서 설정으로 제어 가능한 부분이 아닌 것으로 현재 판단되어서 일단 damp를 사용해서 임의로 값을 증가, 증감시켜 범위 내 변하는 값으로 움직임을 처리한다.
이 값이 필요한 이유는 애니메이션을 블렌딩으로 처리하기 때문에 자연스러운 애니메이션을 표현하기 위해서 시작-도착 값까지의 변화하는 값이 필요하다.
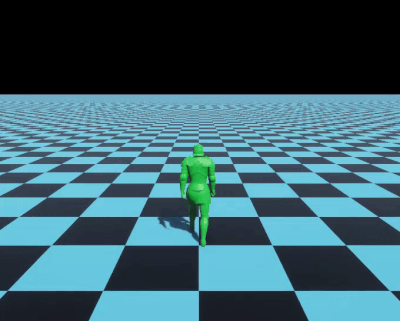
'Develop > Unity' 카테고리의 다른 글
Input System - Player Input (0) | 2025.04.11 |
---|---|
Input System - Input Actions (0) | 2025.04.10 |
유니티 기본 물리 샘플 (0) | 2025.03.21 |
구글 계정 연동 (1) | 2025.02.28 |
2D 애니메이션, 이펙트 (0) | 2024.12.03 |